1711번: 직각삼각형

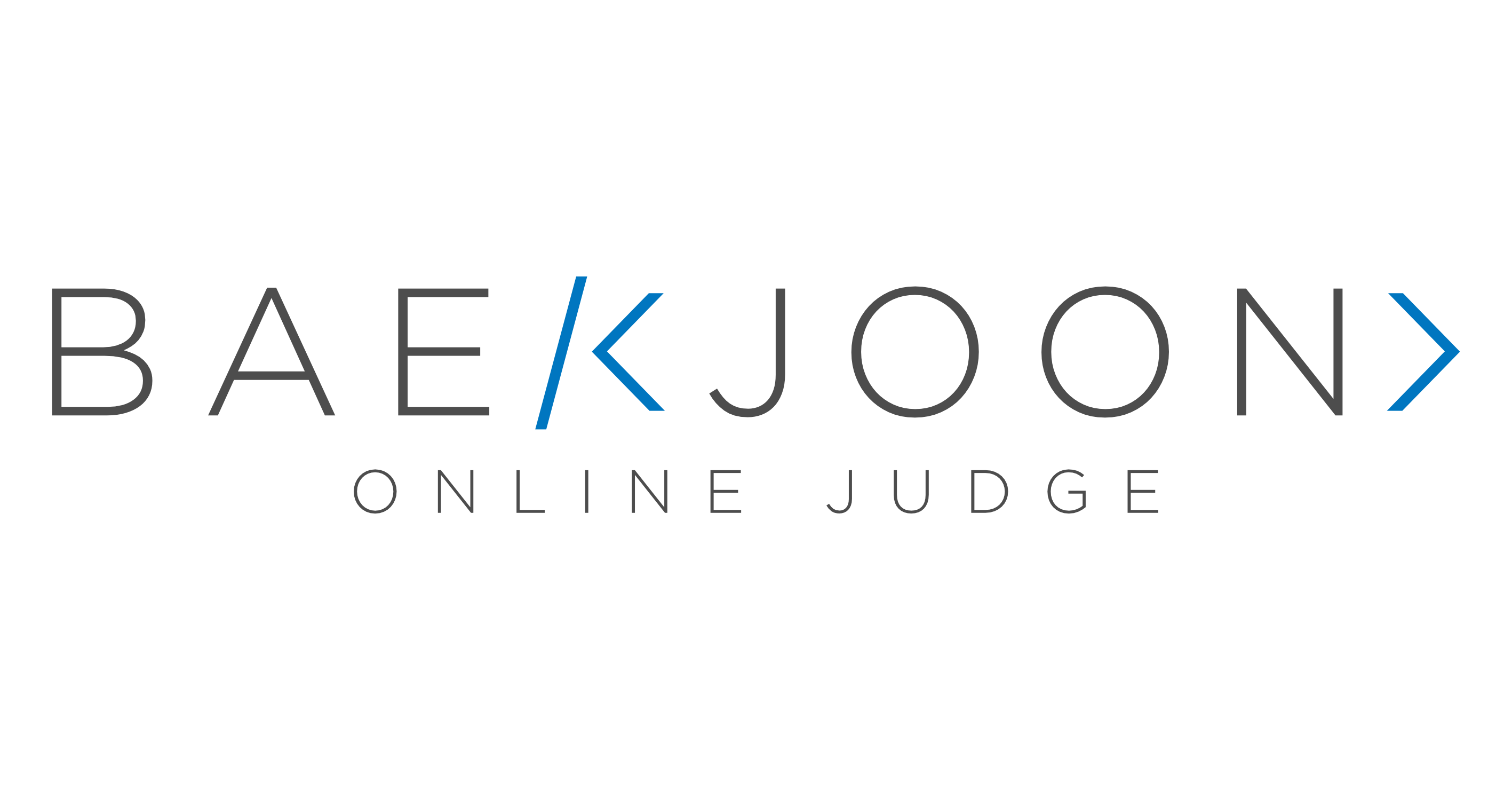
키워드
2차원 평면에 N개의 점이 주어져 있다. 이 중에서 세 점을 골랐을 때, 직각삼각형이 몇 개나 있는지를 구하는 프로그램을 작성하시오.
구현
한 점을 기준으로 다른 두 점을 향한 벡터의 내적이 0이면 직각임을 이용한다.
코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.StringTokenizer;
public class java_1711 {
static int N;
static Pair pairs[];
static class Pair {
int y, x;
public Pair(int y, int x) {
this.y = y;
this.x = x;
}
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
N = Integer.parseInt(br.readLine());
pairs = new Pair[N];
for (int i = 0; i < N; i++) {
StringTokenizer st = new StringTokenizer(br.readLine());
int x = Integer.parseInt(st.nextToken());
int y = Integer.parseInt(st.nextToken());
Pair pair = new Pair(y, x);
pairs[i] = pair;
}
int result = solution();
System.out.println(result);
br.close();
}
// 직각 삼각형의 갯수 반환하는 메소드
private static int solution() {
int count = 0;
//
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (i == j) {
continue;
}
for (int k = j + 1; k < N; k++) {
if (j == k || k == i) {
continue;
}
Pair firstPair = pairs[i];
Pair secondPair = pairs[j];
Pair thirdPair = pairs[k];
Pair firstVector = new Pair(secondPair.y - firstPair.y, secondPair.x - firstPair.x);
Pair secondVector = new Pair(thirdPair.y - firstPair.y, thirdPair.x - firstPair.x);
if ((long) (firstVector.y) * secondVector.y + (long) (firstVector.x) * secondVector.x == 0) {
count++;
}
}
}
}
return count;
}
// 두 점 사이 길이의 제곱 반환 메소드
private static long calcLengthSquare(int first, int second) {
Pair firstPair = pairs[first];
Pair secondPair = pairs[second];
long length = (long) Math.pow(firstPair.x - secondPair.x, 2) + (long) Math.pow(firstPair.y - secondPair.y, 2);
return length;
}
}
Uploaded by N2T
'Coding Test > BOJ' 카테고리의 다른 글
[BOJ/JAVA] 1744번: 수 묶기 (0) | 2023.04.03 |
---|---|
[BOJ/JAVA] 1198번: 삼각형으로 자르기 (0) | 2023.04.03 |
[BOJ/JAVA] 11758번: CCW (0) | 2023.04.03 |
[BOJ/JAVA] 1701번: Cubeditor (0) | 2023.03.20 |
[BOJ/JAVA] 1599번: 민식어 (0) | 2023.03.20 |