
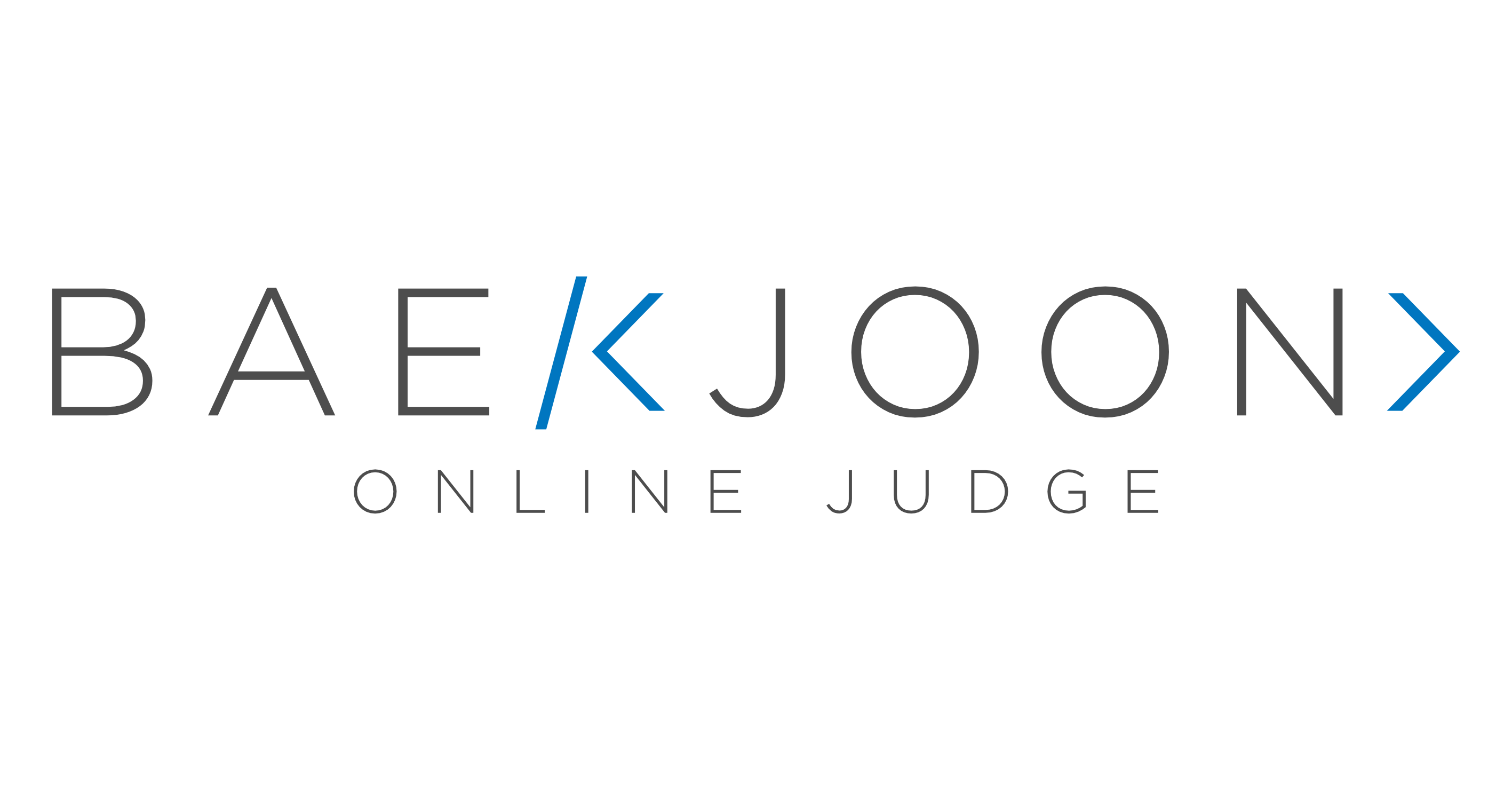
키워드
민식어는 a b k d e g h i l m n ng o p r s t u w y의 순서
ng는 n과 o사이에 오는 하나의 알파벳
민식어의 순서대로 정렬하는 프로그램
구현
다음 3가지를 구현하면 된다.
- 민식어의 우선순위를 처리하는 방법
- ng를 판단하는 방법
- 문자열을 특정 조건으로 정렬하는 방법
1. 민식어의 우선순위 처리하는 방법
final static Map<Character, Integer> minsikMap
민식어는 a b k d e g h i l m n ng o p r s t u w y의 순서대로 우선순위를 가진다.
따라서 해당 문자가 몇 번째 우선순위인지 map에 저장해두도록 하자
ng는 문자열이므로 -라는 문자로 치환하여 저장하자.
2. ng를 판단하는 방법
민식어의 우선순위는 ng를 제외하고 단일 문자이다.
따라서 문자 하나하나를 읽으면서 n다음에 g가 나오는지 판단해야한다.
현재 문자가 n이고, 다음 문자가 존재하면서 g일때 ng라고 판단
한다.
// 현재 알파벳이 ng인지 판단하기
if (o1Index < o1.length() && 'n' == o1Character) {
// 다음 알파벳이 g라면..
if (o1.charAt(o1Index) == 'g') {
o1Character = '-';
o1Index++;
}
}
3. 문자열을 특정 조건으로 정렬하는 방법
Java에서 데이터를 정렬하는 방법은 Comparator, Comparable 2가지 방법이있다.
여기서는 Comparator
를 이용하여 구현을 할 것이다.
Arrays.sort()
메소드는 인자로 Comparator
를 받는데, 이 안에 compare
라는 메서드를 직접 구현하면 해당 우선순위대로 정렬이 되는 방식이다.
기본적인 메서드 구조는 다음과 같다.
public int compare(String o1, String o2)
반환 값이 음수이면 o1이 우선, 반환 값이 양수면 o2가 우선순위를 가진다.
우선순위는 1에서 처리를 했고, ng판단도 2에서 구현을 했으므로 이를 이용하여 정렬한다.
코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.Comparator;
import java.util.Map;
public class java_1599 {
final static Map<Character, Integer> minsikMap = Map.ofEntries(
Map.entry('a', 1),
Map.entry('b', 2),
Map.entry('c', 3),
Map.entry('k', 4),
Map.entry('d', 5),
Map.entry('e', 6),
Map.entry('g', 7),
Map.entry('h', 8),
Map.entry('i', 9),
Map.entry('l', 10),
Map.entry('m', 11),
Map.entry('n', 12),
Map.entry('-', 13),
Map.entry('o', 14),
Map.entry('p', 15),
Map.entry('r', 16),
Map.entry('s', 17),
Map.entry('t', 18),
Map.entry('u', 19),
Map.entry('w', 20),
Map.entry('y', 21));
static int N;
static String words[];
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
N = Integer.parseInt(br.readLine());
words = new String[N];
for (int i = 0; i < N; i++) {
words[i] = br.readLine();
}
solution();
printWords();
br.close();
}
// words 배열을 출력한다.
private static void printWords() {
// System.out.println("========");
for (int i = 0; i < words.length; i++) {
System.out.println(words[i]);
}
// System.out.println("========");
}
// words 배열을 민식어에 따라서 정렬한다.
private static void solution() {
Arrays.sort(words, new Comparator<String>() {
@Override
public int compare(String o1, String o2) {
int o1Index = 0, o2Index = 0;
while (o1Index < o1.length() && o2Index < o2.length()) {
// 현재 인덱스 알파벳 추출
char o1Character = o1.charAt(o1Index++);
char o2Character = o2.charAt(o2Index++);
// 현재 알파벳이 ng인지 판단하기
if (o1Index < o1.length() && 'n' == o1Character) {
// 다음 알파벳이 g라면..
if (o1.charAt(o1Index) == 'g') {
o1Character = '-';
o1Index++;
}
}
if (o2Index < o2.length() && 'n' == o2Character) {
// 다음 알파벳이 g라면..
if (o2.charAt(o2Index) == 'g') {
o2Character = '-';
o2Index++;
}
}
// 현재 알파벳이 같다면.. 계속 비교
if (o1Character == o2Character) {
continue;
}
// 다르면 우선순위 판별
return minsikMap.get(o1Character) - minsikMap.get(o2Character);
}
// 반복문을 빠져나온 경우
// 1. o1이 짧은 문자열인 경우
if (o1Index == o1.length()) {
return -1;
}
// 2. o2가 짧은 문자열인 경우
// 두 문자열이 같은 경우는 존재하지 않음
else {
return 1;
}
}
});
}
}
Uploaded by N2T
'Coding Test > BOJ' 카테고리의 다른 글
[BOJ/JAVA] 11758번: CCW (0) | 2023.04.03 |
---|---|
[BOJ/JAVA] 1701번: Cubeditor (0) | 2023.03.20 |
[BOJ/JAVA] 17609번: 회문 (0) | 2023.03.20 |
[BOJ/JAVA] 1958번: LCS 3 (0) | 2023.03.20 |
[BOJ/JAVA] 11060번: 점프 점프 (0) | 2023.03.14 |